複数のフルカラーLED(マイコン内蔵RGBLED 8mm PL9823-F8)で自作フォント(アルファベット大文字小文字と数字)を表示やスクロールさせます。
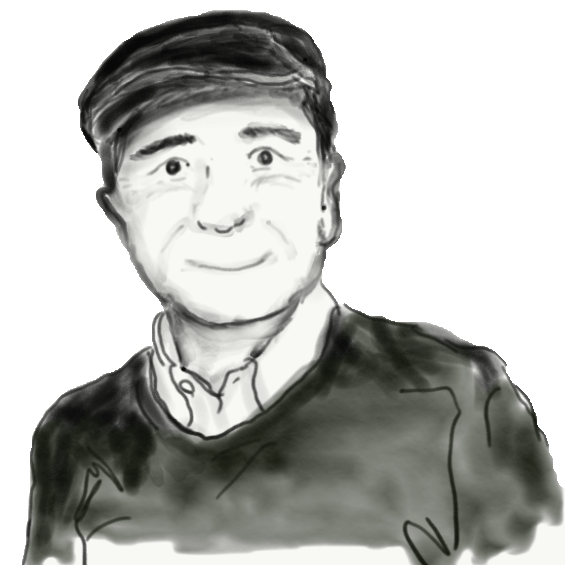
8×8のLEDに自作のフォントを読み込んで文字を表示やスクロールさせます。
フルカラーLED(マイコン内蔵RGBLED 8mm PL9823-F8)マトリックスモジュール
配線図
Vー 38:GND
IN 1:GPIO 0
V+ 40:VBUS
LEDによって配置が変わるので信号線(黄色)と電源を間違えないように。
MicroPythonプログラム
メインプログラム
import neopixel import time from machine import Pin # フォントを設定 import font_basic_8x8size import font_basic_8x5size # LEDの設定 NUM_LEDS = 26 * 8 * 8 # LEDの数 (アルファベットの文字数 × 8) PIN = 0 # データピン # NeoPixelオブジェクトの作成 np = neopixel.NeoPixel(Pin(PIN), NUM_LEDS) colors = { 'off' : (0, 0, 0), 'white': (10, 10, 10), 'red' : (10, 0, 0), 'green': (0, 10, 0), 'blue' : (0, 0, 10), } # LEDにアルファベットを描画する関数 def draw_alphabet(alphabet, form, in_color, out_color): print(form) print(alphabet) if form == '8x5': pattern = font_basic_8x5size.alphabets.get(alphabet, None) elif form == '8x8': pattern = font_basic_8x8size.alphabets.get(alphabet, None) else: return # エラー処理など、未知のフォーマットの場合は何もしない if pattern: for y in range(8): for x in range(8): index = y * 8 + x if pattern[y][x] == '0': np[index] = in_color # 文字の色 else: np[index] = out_color # 背景の色 np.write() # テキストを描画する関数 def draw_text(text, form, in_color_name, out_color_name, speed): in_color = colors.get(in_color_name, (0, 0, 0)) out_color = colors.get(out_color_name, (0, 0, 0)) for letter in text: draw_alphabet(letter, form, in_color, out_color) time.sleep(speed) # 1秒ごとに次の文字に移動 def slide_in_animation(letter, form, in_color, out_color, speed): pattern = font_basic_8x5size.alphabets.get(letter, None) if pattern: # 初期位置は画面外の右端に設定する for x_offset in range(8, -8, -1): # 右端から左端に向かってシフト for y in range(8): for x in range(8): if 0 <= x + x_offset < 8: # 画面内に収まる範囲のLEDのみを操作 index = y * 8 + x + x_offset if pattern[y][x] == '0': np[index] = in_color else: np[index] = out_color else: # 画面外の部分は消灯 np[y * 10 + x] = out_color np.write() time.sleep(0.02) # 表示の速度を調整するための待機時間 def draw_text_with_slide_in(text, form, in_color_name="white", out_color_name="off", speed=0.1): in_color = colors.get(in_color_name, (0, 0, 0)) out_color = colors.get(out_color_name, (0, 0, 0)) for letter in text: print (letter) slide_in_animation(letter, form, in_color, out_color, speed) time.sleep(0.02) # 文字と文字の間に少し待機する # メインループ while True: #draw_text("ailox", "8x5", "red", "off", 0.2) draw_text("0ilox", "8x8", "red", "off", 1.0) #draw_text_with_slide_in("ABCDEF", "8x5", "blue", "off", 0.2)
フォントファイル8x8
# font_basic_8x8size.py alphabets = { 'A': [ " 00", " 000", " 00 0", " 00 0", " 000000", " 00 0", "00 0", " " ], 'B': [ "0000000 ", " 00 00", " 00 00", " 000000 ", " 00 00", " 00 00", "0000000 ", " " ], 'C': [ " 00000 ", " 00 00", "00 ", "00 ", "00 ", " 00 00", " 00000 ", " " ], 'D': [ "000000 ", " 00 00 ", " 00 00", " 00 00", " 00 00", " 00 00 ", "000000 ", " " ], 'E': [ "00000000", " 00 0", " 00 ", " 000000 ", " 00 ", " 00 0", "00000000", " " ], 'F': [ "00000000", " 00 0", " 00 ", " 000000 ", " 00 ", " 00 ", "0000 ", " " ], 'G': [ " 00000 ", " 00 00", "00 ", "00 0000", "00 00", " 00 000", " 0000 0", " " ], 'H': [ "000 000", " 00 00 ", " 00 00 ", " 000000 ", " 00 00 ", " 00 00 ", "000 000", " " ], 'I': [ " 000000 ", " 00 ", " 00 ", " 00 ", " 00 ", " 00 ", " 000000 ", " " ], 'J': [ " 0000", " 00 ", " 00 ", "000 00 ", "00 00 ", "00 00 ", " 00000 ", " " ], 'K': [ "000 00", " 00 00 ", " 00 00 ", " 0000 ", " 00 00 ", " 00 00 ", "000 00", " " ], 'L': [ "0000 ", " 00 ", " 00 ", " 00 ", " 00 ", " 00 0", "00000000", " " ], 'M': [ "00 00", "000 000", "00 00 00", "00 00", "00 00", "00 00", "00 00", " " ], 'N': [ "000 0000", " 00 00 ", " 000 00 ", " 00 000 ", " 00 00 ", " 00 00 ", "0000 000", " " ], 'O': [ " 0000 ", " 00 00 ", "000 000", "000 000", "000 000", " 00 00 ", " 0000 ", " " ], 'P': [ "0000000 ", " 00 00", " 00 00", " 000000 ", " 00 ", " 00 ", "0000 ", " " ], 'Q': [ " 0000 ", " 00 00 ", "000 000", "000 000", "000 0000", " 00 00 ", " 000 00", " " ], 'R': [ "0000000 ", " 00 00", " 00 00", " 000000 ", " 00 00 ", " 00 00 ", "0000 00", " " ], 'S': [ " 000000 ", "00 00", "000 ", " 0000 ", " 000", "00 00", " 000000 ", " " ], 'T': [ "00000000", "0 00 0 ", " 00 ", " 00 ", " 00 ", " 00 ", " 0000 ", " " ], 'U': [ "00 00", "00 00", "00 00", "00 00", "00 00", "00 00", " 000000 ", " " ], 'V': [ "00 00", "00 00", "00 00", "00 00", " 00 00 ", " 0000 ", " 00 ", " " ], 'W': [ "00 00", "00 00", "00 00", "00 00", "00 00 00", "000 000", "00 00", " " ], 'X': [ "00 00", "00 00", " 00 00 ", " 0000 ", " 00 00 ", "00 00", "00 00", " " ], 'Y': [ "00 00", "00 00", " 00 00 ", " 0000 ", " 00 ", " 00 ", " 0000 ", " " ], 'Z': [ "0000000 ", "0 00 ", " 00 ", " 00 ", " 00 ", " 00 0", "00000000", " " ], 'a': [ " ", " ", " 0000 ", " 0 ", " 00000 ", " 0 0 ", " 00000 ", " " ], 'b': [ " 0 ", " 0 ", " 0 000 ", " 00 0 ", " 0 0 ", " 0 0 ", " 000000 ", " " ], 'c': [ " ", " ", " 0000 ", " 0 ", " 0 ", " 0 ", " 0000 ", " " ], 'd': [ " 0 ", " 0 ", " 000 0 ", " 0 00 ", " 0 0 ", " 0 0 ", " 00000 ", " " ], 'e': [ " ", " ", " 0000 ", " 0 0 ", " 000000 ", " 0 ", " 0000 ", " " ], 'f': [ " 000 ", " 0 ", " 000000 ", " 0 ", " 0 ", " 0 ", " 0 ", " " ], 'g': [ " ", " ", " 00000 ", " 0 0 ", " 00000 ", " 0 ", " 0 0 ", " 0000 " ], 'h': [ " 0 ", " 0 ", " 0 000 ", " 00 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " " ], 'i': [ " 0 ", " ", " 00 ", " 0 ", " 0 ", " 0 ", " 000 ", " " ], 'j': [ " 0 ", " ", " 00 ", " 0 ", " 0 ", " 0 ", " 0 0 ", " 00 " ], 'k': [ " 0 ", " 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 00 0 ", " 0 0 ", " " ], 'l': [ " ", " 00 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0000 ", " " ], 'm': [ " ", " ", " 00 00 ", " 0 0 0 ", " 0 0 0 ", " 0 0 0 ", " 0 0 0 ", " " ], 'n': [ " ", " ", " 0 000 ", " 00 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " " ], 'o': [ " ", " ", " 0000 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0000 ", " " ], 'p': [ " ", " ", " 0 000 ", " 0 0 ", " 0 0 ", " 00000 ", " 0 ", " 0 " ], 'q': [ " ", " ", " 000 0 ", " 0 00 ", " 0 0 ", " 00000 ", " 0 ", " 0 " ], 'r': [ " ", " ", " 0 000 ", " 00 0 ", " 0 ", " 0 ", " 0 ", " " ], 's': [ " ", " ", " 0000 ", " 0 ", " 0000 ", " 0 ", " 0000 ", " " ], 't': [ " 0 ", " 0 ", " 0000 ", " 0 ", " 0 ", " 0 ", " 00 ", " " ], 'u': [ " ", " ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 00 ", " 000 0 ", " " ], 'v': [ " ", " ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 00 ", " " ], 'w': [ " ", " ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 00 0 ", " 0 0 ", " " ], 'x': [ " ", " ", " 0 0 ", " 0 0 ", " 00 ", " 0 0 ", " 0 0 ", " " ], 'y': [ " ", " ", " 0 0 ", " 0 0 ", " 0 0 ", " 00000 ", " 0 ", " 0000 " ], 'z': [ " ", " ", " 00000 ", " 0 ", " 0 ", " 0 ", " 00000 ", " " ], '0': [ " 0000 ", " 0 0 ", " 0 00 ", " 0 0 0 ", " 0 0 0 ", " 00 0 ", " 0000 ", " " ], '1': [ " 0 ", " 00 ", " 0 ", " 0 ", " 0 ", " 0 ", " 000 ", " " ], '2': [ " 0000 ", " 0 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 000000 ", " " ], '3': [ " 0000 ", " 0 0 ", " 0 ", " 000 ", " 0 ", " 0 0 ", " 0000 ", " " ], '4': [ " 0 ", " 00 ", " 0 0 ", " 0 0 ", " 000000 ", " 0 ", " 0 ", " " ], '5': [ " 000000 ", " 0 ", " 0 ", " 00000 ", " 0 ", " 0 0 ", " 0000 ", " " ], '6': [ " 0000 ", " 0 0 ", " 0 ", " 00000 ", " 0 0 ", " 0 0 ", " 0000 ", " " ], '7': [ " 000000 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " " ], '8': [ " 0000 ", " 0 0 ", " 0 0 ", " 0000 ", " 0 0 ", " 0 0 ", " 0000 ", " " ], '9': [ " 0000 ", " 0 0 ", " 0 0 ", " 00000 ", " 0 ", " 0 0 ", " 0000 ", " " ], ' ': [ " ", " ", " ", " ", " ", " ", " ", " " ] }
フォントファイル8×5
# font_basic_8x5size.py alphabets = { 'A': [ " 000 ", "0 0 ", "0 0 ", "00000 ", "0 0 ", "0 0 ", "0 0 ", " " ], 'B': [ "0000 ", "0 0 ", "0 0 ", "0000 ", "0 0 ", "0 0 ", "0000 ", " " ], 'C': [ " 000 ", "0 0 ", "0 ", "0 ", "0 ", "0 0 ", " 000 ", " " ], 'D': [ " 0000 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0000 ", " " ], 'E': [ " 000000 ", " 0 ", " 0 ", " 0000 ", " 0 ", " 0 ", " 000000 ", " " ], 'F': [ " 000000 ", " 0 ", " 0 ", " 0000 ", " 0 ", " 0 ", " 0 ", " " ], 'G': [ " 0000 ", " 0 0 ", " 0 ", " 0 ", " 0 000 ", " 0 0 ", " 0000 ", " " ], 'H': [ " 0 0 ", " 0 0 ", " 0 0 ", " 000000 ", " 0 0 ", " 0 0 ", " 0 0 ", " " ], 'I': [ " 0000 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0000 ", " " ], 'J': [ " 000 ", " 0 ", " 0 ", " 0 ", " 0 0 ", " 0 0 ", " 000 ", " " ], 'K': [ " 0 0 ", " 0 0 ", " 0 0 ", " 00 ", " 0 0 ", " 0 0 ", " 0 0 ", " " ], 'L': [ " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 000000 ", " " ], 'M': [ " 0 0 ", " 00 00 ", " 0 00 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " " ], 'N': [ " 0 0 ", " 00 0 ", " 0 0 0 ", " 0 0 0 ", " 0 00 ", " 0 0 ", " 0 0 ", " " ], 'O': [ " 0000 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0000 ", " " ], 'P': [ " 00000 ", " 0 0 ", " 0 0 ", " 00000 ", " 0 ", " 0 ", " 0 ", " " ], 'Q': [ " 0000 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 0 ", " 00000 ", " 0" ], 'R': [ " 00000 ", " 0 0 ", " 0 0 ", " 00000 ", " 0 0 ", " 0 0 ", " 0 0 ", " " ], 'S': [ " 0000 ", " 0 0 ", " 0 ", " 0000 ", " 0 ", " 0 0 ", " 0000 ", " " ], 'T': [ " 000000 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " " ], 'U': [ " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0000 ", " " ], 'V': [ " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 00 ", " " ], 'W': [ " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 00 0 ", " 00 00 ", " 0 0 ", " " ], '0': [ " 0 0 ", " 0 0 ", " 0 0 ", " 00 ", " 0 0 ", " 0 0 ", " 0 0 ", " " ], 'Y': [ " 0 0 ", " 0 0 ", " 0 0 ", " 00 ", " 0 ", " 0 ", " 0 ", " " ], 'Z': [ " 000000 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 000000 ", " " ], 'a': [ " ", " ", " 0000 ", " 0 ", " 00000 ", " 0 0 ", " 00000 ", " " ], 'b': [ " 0 ", " 0 ", " 0 000 ", " 00 0 ", " 0 0 ", " 0 0 ", " 000000 ", " " ], 'c': [ " ", " ", " 0000 ", " 0 ", " 0 ", " 0 ", " 0000 ", " " ], 'd': [ " 0 ", " 0 ", " 000 0 ", " 0 00 ", " 0 0 ", " 0 0 ", " 00000 ", " " ], 'e': [ " ", " ", " 0000 ", " 0 0 ", " 000000 ", " 0 ", " 0000 ", " " ], 'f': [ " 000 ", " 0 ", " 000000 ", " 0 ", " 0 ", " 0 ", " 0 ", " " ], 'g': [ " ", " ", " 00000 ", " 0 0 ", " 00000 ", " 0 ", " 0 0 ", " 0000 " ], 'h': [ " 0 ", " 0 ", " 0 000 ", " 00 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " " ], 'i': [ " 0 ", " ", " 00 ", " 0 ", " 0 ", " 0 ", " 000 ", " " ], 'j': [ " 0 ", " ", " 00 ", " 0 ", " 0 ", " 0 ", " 0 0 ", " 00 " ], 'k': [ " 0 ", " 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 00 0 ", " 0 0 ", " " ], 'l': [ " 00 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 00 ", " " ], 'm': [ " ", " ", " 00 00 ", " 0 0 0 ", " 0 0 0 ", " 0 0 0 ", " 0 0 0 ", " " ], 'n': [ " ", " ", " 0 000 ", " 00 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " " ], 'o': [ " ", " ", " 0000 ", " 0 0 ", " 0 0 ", " 0 0 ", " 0000 ", " " ], 'p': [ " ", " ", " 0 000 ", " 0 0 ", " 0 0 ", " 00000 ", " 0 ", " 0 " ], 'q': [ " ", " ", " 000 0 ", " 0 00 ", " 0 0 ", " 00000 ", " 0 ", " 0 " ], 'r': [ " ", " ", " 0 000 ", " 00 0 ", " 0 ", " 0 ", " 0 ", " " ], 's': [ " ", " ", " 0000 ", " 0 ", " 0000 ", " 0 ", " 0000 ", " " ], 't': [ " 0 ", " 0 ", " 0000 ", " 0 ", " 0 ", " 0 ", " 00 ", " " ], 'u': [ " ", " ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 00 ", " 000 0 ", " " ], 'v': [ " ", " ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 0 ", " 00 ", " " ], 'w': [ " ", " ", " 0 0 ", " 0 0 ", " 0 0 ", " 0 00 0 ", " 0 0 ", " " ], 'x': [ " ", " ", " 0 0 ", " 0 0 ", " 00 ", " 0 0 ", " 0 0 ", " " ], 'y': [ " ", " ", " 0 0 ", " 0 0 ", " 0 0 ", " 00000 ", " 0 ", " 0000 " ], 'z': [ " ", " ", " 00000 ", " 0 ", " 0 ", " 0 ", " 00000 ", " " ], '0': [ " 0000 ", " 0 0 ", " 0 00 ", " 0 0 0 ", " 0 0 0 ", " 00 0 ", " 0000 ", " " ], '1': [ " 0 ", " 00 ", " 0 ", " 0 ", " 0 ", " 0 ", " 000 ", " " ], '2': [ " 0000 ", " 0 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 000000 ", " " ], '3': [ " 0000 ", " 0 0 ", " 0 ", " 000 ", " 0 ", " 0 0 ", " 0000 ", " " ], '4': [ " 0 ", " 00 ", " 0 0 ", " 0 0 ", " 000000 ", " 0 ", " 0 ", " " ], '5': [ " 000000 ", " 0 ", " 0 ", " 00000 ", " 0 ", " 0 0 ", " 0000 ", " " ], '6': [ " 0000 ", " 0 0 ", " 0 ", " 00000 ", " 0 0 ", " 0 0 ", " 0000 ", " " ], '7': [ " 000000 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " 0 ", " " ], '8': [ " 0000 ", " 0 0 ", " 0 0 ", " 0000 ", " 0 0 ", " 0 0 ", " 0000 ", " " ], '9': [ " 0000 ", " 0 0 ", " 0 0 ", " 00000 ", " 0 ", " 0 0 ", " 0000 ", " " ], ' ': [ " ", " ", " ", " ", " ", " ", " ", " " ] }
使い方
補足
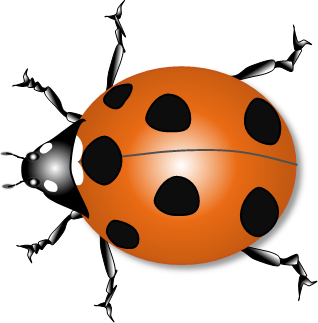
テンテン
このプログラムとフォントは教室の生徒の作成したものです。
使用の際はご連絡ください。